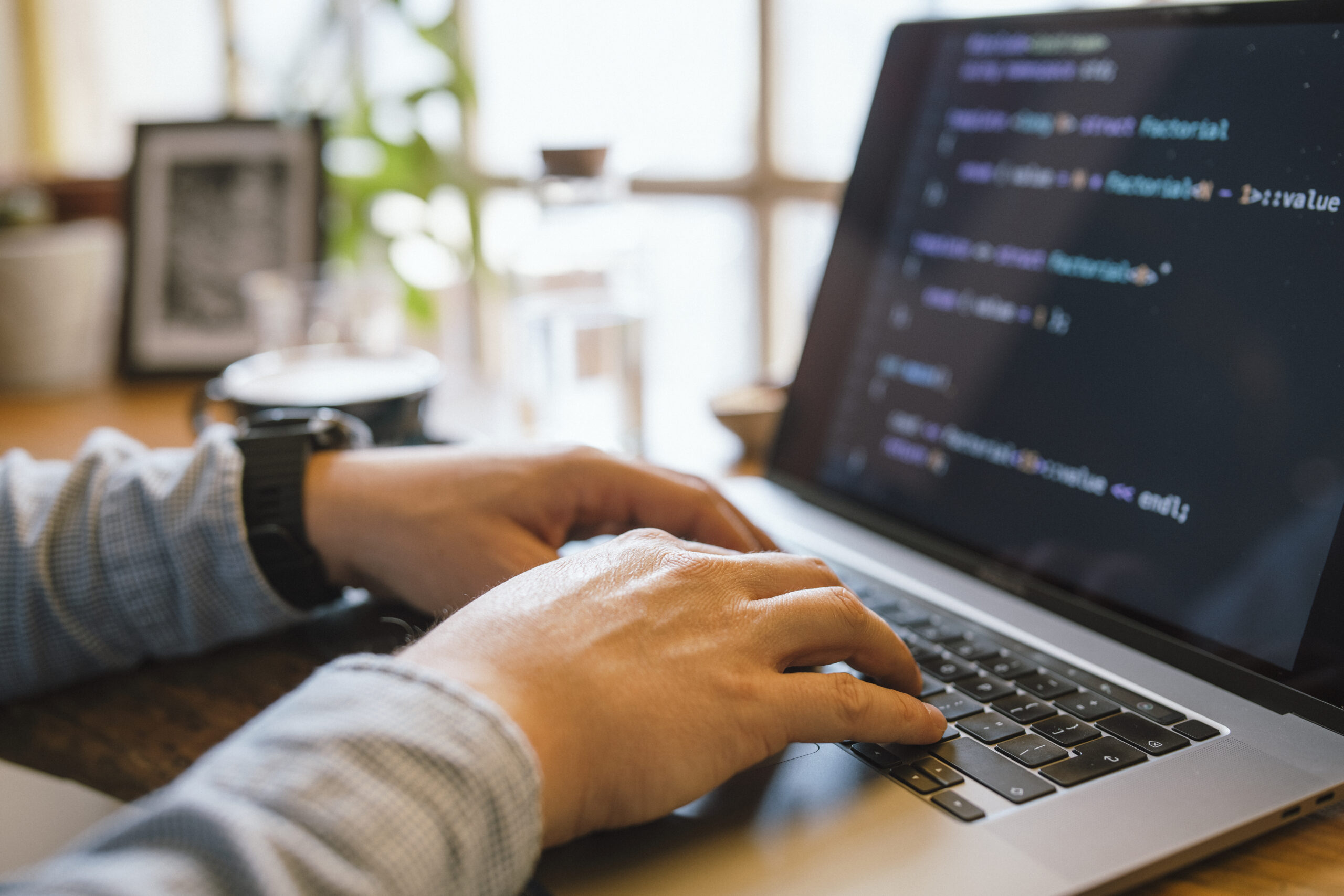
Debugging is Probably the most crucial — nonetheless often disregarded — expertise in the developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about understanding how and why points go Incorrect, and Understanding to Feel methodically to resolve troubles proficiently. Whether or not you're a beginner or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of stress and substantially transform your productiveness. Allow me to share many approaches to aid builders degree up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
On the list of fastest ways builders can elevate their debugging competencies is by mastering the instruments they use every single day. Although creating code is one particular Portion of improvement, knowing tips on how to communicate with it successfully for the duration of execution is equally vital. Modern-day advancement environments come Geared up with effective debugging capabilities — but many builders only scratch the surface area of what these applications can do.
Take, for instance, an Integrated Development Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools assist you to established breakpoints, inspect the worth of variables at runtime, step by code line by line, and in some cases modify code around the fly. When made use of accurately, they Enable you to observe exactly how your code behaves during execution, which happens to be priceless for monitoring down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, watch community requests, see authentic-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can transform discouraging UI issues into manageable jobs.
For backend or procedure-degree developers, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate over working procedures and memory administration. Learning these instruments may have a steeper Studying curve but pays off when debugging effectiveness problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into comfortable with Variation control methods like Git to grasp code heritage, obtain the precise second bugs ended up launched, and isolate problematic variations.
Finally, mastering your tools indicates going outside of default configurations and shortcuts — it’s about acquiring an personal expertise in your development environment to ensure that when difficulties occur, you’re not missing in the dead of night. The higher you already know your instruments, the greater time you can spend solving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the Problem
One of the most significant — and infrequently forgotten — methods in powerful debugging is reproducing the trouble. In advance of jumping in to the code or making guesses, builders need to have to make a constant surroundings or scenario where by the bug reliably seems. Without having reproducibility, fixing a bug results in being a activity of chance, normally resulting in wasted time and fragile code improvements.
Step one in reproducing a problem is accumulating just as much context as you can. Ask issues like: What actions triggered The problem? Which environment was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it gets to be to isolate the exact problems under which the bug happens.
When you’ve gathered sufficient facts, seek to recreate the trouble in your neighborhood setting. This could indicate inputting the same knowledge, simulating equivalent person interactions, or mimicking method states. If The problem seems intermittently, contemplate crafting automated exams that replicate the edge situations or point out transitions involved. These assessments don't just aid expose the situation but in addition reduce regressions in the future.
Often, The difficulty may be surroundings-precise — it might materialize only on particular running units, browsers, or under certain configurations. Working with applications like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is usually instrumental in replicating such bugs.
Reproducing the trouble isn’t only a action — it’s a mentality. It needs endurance, observation, and also a methodical solution. But once you can consistently recreate the bug, you're currently midway to correcting it. That has a reproducible state of affairs, You may use your debugging tools more successfully, examination opportunity fixes properly, and communicate much more clearly together with your group or consumers. It turns an summary grievance into a concrete challenge — and that’s where developers prosper.
Examine and Comprehend the Mistake Messages
Mistake messages in many cases are the most worthy clues a developer has when a thing goes Mistaken. As an alternative to viewing them as annoying interruptions, developers must discover to take care of mistake messages as direct communications in the technique. They normally inform you what exactly occurred, where it transpired, and often even why it occurred — if you know the way to interpret them.
Start by examining the concept cautiously As well as in entire. Numerous builders, particularly when beneath time stress, look at the initial line and immediately start out producing assumptions. But further while in the error stack or logs may lie the genuine root trigger. Don’t just duplicate and paste error messages into search engines — browse and realize them very first.
Crack the error down into sections. Is it a syntax mistake, a runtime exception, or a logic mistake? Will it place to a specific file and line range? What module or function activated it? These questions can guidebook your investigation and issue you toward the dependable code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically abide by predictable patterns, and Finding out to recognize these can considerably speed up your debugging method.
Some glitches are imprecise or generic, and in All those cases, it’s important to look at the context by which the error happened. Look at associated log entries, input values, and up to date variations while in the codebase.
Don’t forget compiler or linter warnings possibly. These often precede greater troubles and supply hints about opportunity bugs.
Ultimately, error messages are usually not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint troubles speedier, cut down debugging time, and turn into a more productive and self-confident developer.
Use Logging Correctly
Logging is Among the most highly effective applications inside of a developer’s debugging toolkit. When utilized successfully, it provides true-time insights into how an software behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or phase with the code line by line.
An excellent logging tactic commences with being aware of what to log and at what degree. Frequent logging amounts consist of DEBUG, INFO, Alert, Mistake, and Lethal. Use DEBUG for in-depth diagnostic facts through growth, Data for common occasions (like successful get started-ups), WARN for opportunity difficulties that don’t split the application, Mistake for true troubles, and Deadly when the method can’t continue.
Prevent flooding your logs with extreme or irrelevant information. Too much logging can obscure significant messages and slow down your system. Center on critical functions, state improvements, input/output values, and important determination points as part of your code.
Format your log messages Obviously and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s simpler to trace issues in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all devoid of halting the program. They’re Specially valuable in production environments in which stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, clever logging is about balance and clarity. Which has a nicely-considered-out logging approach, you'll be able to lessen the time it takes to spot troubles, attain deeper visibility into your apps, and Increase the General maintainability and dependability of your respective code.
Imagine Like a Detective
Debugging is not only a complex endeavor—it's a kind of investigation. To proficiently identify and repair bugs, developers have to approach the process like a detective solving a secret. This mentality helps break down sophisticated troubles into workable sections and observe clues logically to uncover the foundation cause.
Commence by collecting evidence. Consider the indicators of the situation: mistake messages, incorrect output, or effectiveness difficulties. Identical to a detective surveys against the law scene, collect as much relevant information as you are able to with out jumping to conclusions. Use logs, test conditions, and person stories to piece together a transparent photograph of what’s occurring.
Following, kind hypotheses. Request by yourself: What may be leading to this conduct? Have any modifications not too long ago been created towards the codebase? Has this issue happened in advance of beneath equivalent circumstances? The intention will be to slender down opportunities and recognize possible culprits.
Then, exam your theories systematically. Endeavor to recreate the challenge inside a managed setting. Should you suspect a specific functionality or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, ask your code issues and Allow the effects direct you nearer to the truth.
Pay out shut consideration to little aspects. Bugs usually disguise from the least envisioned areas—similar to a lacking semicolon, an off-by-1 mistake, or perhaps a race affliction. Be thorough and client, resisting the urge to patch the issue devoid of totally knowledge it. Temporary fixes may conceal the actual issue, just for it to resurface afterwards.
Finally, retain notes on Everything you tried out and learned. Just as detectives log their investigations, documenting your debugging process can preserve time for upcoming problems and enable others realize your reasoning.
By imagining like a detective, developers can sharpen their analytical capabilities, tactic troubles methodically, and come to be more effective at uncovering hidden troubles in advanced systems.
Compose Assessments
Crafting checks is one of the most effective solutions to help your debugging abilities and All round growth effectiveness. Assessments don't just assistance capture bugs early but also serve as a safety Internet that provides you self confidence when building improvements towards your codebase. A perfectly-analyzed software is much easier to debug mainly Gustavo Woltmann coding because it helps you to pinpoint exactly in which and when an issue occurs.
Start with unit tests, which focus on individual capabilities or modules. These compact, isolated checks can promptly expose irrespective of whether a selected bit of logic is Doing work as predicted. Whenever a check fails, you right away know in which to appear, significantly reducing some time spent debugging. Device assessments are Specifically helpful for catching regression bugs—issues that reappear just after Earlier currently being set.
Next, combine integration exams and finish-to-stop tests into your workflow. These enable be certain that different parts of your software perform together efficiently. They’re specifically useful for catching bugs that come about in intricate programs with numerous parts or companies interacting. If some thing breaks, your assessments can let you know which Element of the pipeline failed and less than what problems.
Writing assessments also forces you to Imagine critically about your code. To check a function thoroughly, you will need to understand its inputs, predicted outputs, and edge instances. This standard of comprehending Obviously prospects to higher code construction and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug can be a strong starting point. Once the examination fails continuously, you are able to center on correcting the bug and view your exam pass when The problem is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In short, creating assessments turns debugging from the disheartening guessing sport into a structured and predictable system—assisting you catch far more bugs, speedier and more reliably.
Consider Breaks
When debugging a difficult situation, it’s uncomplicated to be immersed in the condition—staring at your screen for hours, hoping Alternative after solution. But Probably the most underrated debugging resources is just stepping absent. Getting breaks will help you reset your head, lower annoyance, and infrequently see The difficulty from the new standpoint.
If you're much too near the code for far too very long, cognitive exhaustion sets in. You would possibly start out overlooking evident glitches or misreading code that you just wrote just hrs earlier. Within this condition, your brain becomes less economical at challenge-fixing. A short walk, a espresso split, and even switching to a special task for ten–quarter-hour can refresh your concentration. A lot of developers report finding the foundation of a difficulty after they've taken time to disconnect, permitting their subconscious operate within the background.
Breaks also enable avert burnout, Specifically during for a longer period debugging classes. Sitting before a display screen, mentally stuck, is don't just unproductive but in addition draining. Stepping away allows you to return with renewed Electricity as well as a clearer mindset. You may perhaps abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you prior to.
For those who’re caught, a very good guideline should be to set a timer—debug actively for forty five–60 minutes, then have a 5–10 moment break. Use that point to move all around, stretch, or do a thing unrelated to code. It might sense counterintuitive, Particularly underneath tight deadlines, nonetheless it actually contributes to a lot quicker and simpler debugging In the long term.
In brief, getting breaks is not a sign of weak point—it’s a smart tactic. It presents your brain Room to breathe, increases your perspective, and aids you steer clear of the tunnel vision that often blocks your progress. Debugging is often a psychological puzzle, and rest is a component of fixing it.
Master From Every single Bug
Each individual bug you experience is much more than simply A short lived setback—It is really an opportunity to increase for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can instruct you something useful in case you take the time to reflect and evaluate what went Improper.
Start off by inquiring on your own a handful of vital questions once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with far better procedures like unit testing, code testimonials, or logging? The solutions typically reveal blind spots within your workflow or knowing and enable you to Construct more powerful coding behavior shifting forward.
Documenting bugs can also be an excellent pattern. Continue to keep a developer journal or manage a log in which you Notice down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring difficulties or widespread blunders—which you can proactively steer clear of.
In team environments, sharing Anything you've uncovered from a bug with your friends might be Specifically potent. Whether it’s through a Slack information, a short write-up, or A fast information-sharing session, helping Many others stay away from the identical issue boosts staff efficiency and cultivates a much better Finding out culture.
Additional importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Instead of dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, some of the finest developers will not be those who compose fantastic code, but individuals who continuously understand from their issues.
Ultimately, Each individual bug you resolve provides a new layer to the talent set. So future time you squash a bug, take a second to replicate—you’ll come away a smarter, additional able developer as a result of it.
Summary
Improving upon your debugging expertise can take time, practice, and persistence — although the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The following time you might be knee-deep inside a mysterious bug, bear in mind: debugging isn’t a chore — it’s a possibility to be better at Everything you do.